4drian
[Mentally Stable]
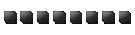 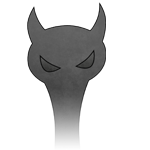
Status: Offline (since 06-11-2009 17:32)
Joined: 07 Sep 2009
Posts: 10, Topics: 6
Location: Romania
Reputation: 91.7   Votes: 4
|
0 0
|
4drian
[Mentally Stable]
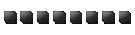 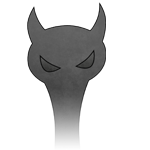
Status: Offline (since 06-11-2009 17:32)
Joined: 07 Sep 2009
Posts: 10, Topics: 6
Location: Romania
Reputation: 91.7   Votes: 4
|
0 0
|
*0ranGe ! extrem
[I ❤ MY POLO!]
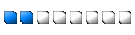 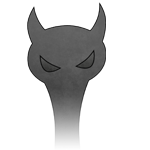
Status: Offline (since 11-12-2017 12:06)
Joined: 01 Jul 2007
Posts: 11419, Topics: 191
Location: Romania
Reputation: 683.4   Votes: 117
|
0 0
|
4drian
[Mentally Stable]
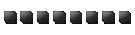 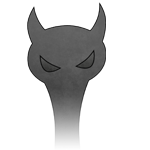
Status: Offline (since 06-11-2009 17:32)
Joined: 07 Sep 2009
Posts: 10, Topics: 6
Location: Romania
Reputation: 91.7   Votes: 4
|
0 0
|