tenegabi
[Freakazoid]
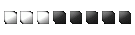 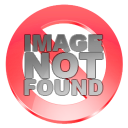
Status: Offline (since 09-05-2022 16:18)
Joined: 09 Oct 2010
Posts: 1794, Topics: 68
Location: Maldaeni
Reputation: 42.2   Votes: 35
|
0 0
|
sTbA
[Dev C# && C++]
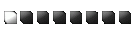 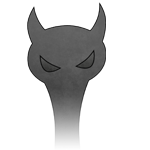
Status: Offline (since 19-08-2022 13:09)
Joined: 15 Dec 2012
Posts: 963, Topics: 71
Location: United Kingdom
Reputation: 349.6   Votes: 52
|
0 0
|
tenegabi
[Freakazoid]
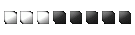 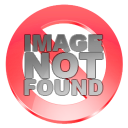
Status: Offline (since 09-05-2022 16:18)
Joined: 09 Oct 2010
Posts: 1794, Topics: 68
Location: Maldaeni
Reputation: 42.2   Votes: 35
|
0 0
|